16
03Simple File Manager Using Php Mysql
- $_FILES["file"]["name"] : indicates name of the uploaded file
- $_FILES["file"]["type"] : indicates type of the uploaded file
- $_FILES["file"]["size"] : indicates size of the uploaded file in bytes
- $_FILES["file"]["tmp_name"] : indicates the file to be stored on temporary server
- $_FILES["file"]["error"] : indicates the error in the file
Generally the default maximum upload file size less than 8mb.Increase file size upload limit using php.ini or htaccess
Any php web application or server configured with default values set in php.ini and .htacess. Generally almost web hosting providers configures the web application to optimum settings, which effects server bandwidth, server memory limit, server disk space, and peak security measures. For file uploading and PHP script execution there is default configuration in PHP.ini. However almost hosting providers give chance to developer to customize this default configuration by override php.ini or .htaccess . some settings can be configured by ini_set() method at run time of script.
Increasing file upload size by php.ini:
upload_max_filesize = 10M ; post_max_size = 20M ; memory_limit = 128M
Increasing file upload size by php.ini:
php_value upload_max_filesize 10M php_value post_max_size 20M php_value memory_limit 128M
Copy the above settings into your .htaccess file and put it in your web root directory. Almost all web host providers give to override the .htacces ,so you can use above method.
HTML form input:
Database Connection:
mysql_select_db('filemgr',mysql_connect('localhost','root',''))or die(mysql_error());
File upload with restrictions:
if(isset($_POST['submit'])!=""){ $name=$_FILES['photo']['name']; $size=$_FILES['photo']['size']; $type=$_FILES['photo']['type']; $temp=$_FILES['photo']['tmp_name']; $error = $_FILES['photo']['error']; $date = date('Y-m-d H:i:s'); if ($error > 0) //Check file upload has error { $_SESSION['alert'] = "danger"; $_SESSION['result'] = "Error: " . $error . "
"; //Sesssio to carry error } else{ if ($size > 500000) { //Check File Size $_SESSION['alert'] = "danger"; $_SESSION['result'] = "Error: Maximum file size 5MB!!!
"; }else{ $targetPath = "files/".$name; if(file_exists($targetPath)){ // Check whether the file name already exist in the server. $rename_file= rand(01,1000).$name; //Rename the current file $targetPath = "files/".$rename_file; }else{ $rename_file = $name; } move_uploaded_file($temp,$targetPath); $query=$conn->query("INSERT INTO upload (name,rename_file,date) VALUES ('$name','$rename_file','$date')"); if($query){ $_SESSION['alert'] = "success"; $_SESSION['result'] = "Well done! File successfully uploaded!!!"; //Session to carry success message } else{ die(mysql_error()); } } } }
Download File in PHP:
"application/pdf", "txt" => "text/plain", "html" => "text/html", "htm" => "text/html", "exe" => "application/octet-stream", "zip" => "application/zip", "doc" => "application/msword", "xls" => "application/vnd.ms-excel", "ppt" => "application/vnd.ms-powerpoint", "gif" => "image/gif", "png" => "image/png", "jpeg" => "image/jpg", "jpg" => "image/jpg", "php" => "text/plain" ); if ($mime_type == '') { echo $file_extension = pathinfo($file, PATHINFO_EXTENSION); if (array_key_exists($file_extension, $known_mime_types)) { $mime_type = $known_mime_types[$file_extension]; } else { $mime_type = "application/force-download"; } ; } ; @ob_end_clean(); if (ini_get('zlib.output_compression')) ini_set('zlib.output_compression', 'Off'); header('Content-Type: ' . $mime_type); header('Content-Disposition: attachment; filename="' . $name . '"'); header("Content-Transfer-Encoding: binary"); header('Accept-Ranges: bytes'); header("Cache-control: private"); header('Pragma: private'); header("Expires: Mon, 26 Jul 2017 05:00:00 GMT"); if (isset($_SERVER['HTTP_RANGE'])) { list($a, $range) = explode("=", $_SERVER['HTTP_RANGE'], 2); list($range) = explode(",", $range, 2); list($range, $range_end) = explode("-", $range); $range = intval($range); if (!$range_end) { $range_end = $size - 1; } else { $range_end = intval($range_end); } $new_length = $range_end - $range + 1; header("HTTP/1.1 206 Partial Content"); header("Content-Length: $new_length"); header("Content-Range: bytes $range-$range_end/$size"); } else { $new_length = $size; header("Content-Length: " . $size); } $chunksize = 1 * (1024 * 1024); $bytes_send = 0; if ($file = fopen($file, 'r')) { if (isset($_SERVER['HTTP_RANGE'])) fseek($file, $range); while (!feof($file) && (!connection_aborted()) && ($bytes_send < $new_length)) { $buffer = fread($file, $chunksize); print($buffer); flush(); $bytes_send += strlen($buffer); } fclose($file); } else die('Error - cannot open file.'); die(); } set_time_limit(0); $file_path = 'files/' . $_REQUEST['filename']; output_file($file_path, '' . $_REQUEST['filename'] . '', ''); ?>
By Thirumani Raj posted on - 16th Mar 2017
Social Oauth Login
Personalized Map Navigation
Online Image Compression Tool
Image CompressionAdvertisement
Recent Posts
- « Personalized Map Navigation
- « Remjs solves the problem of mobile terminal adaptation
- « Picture centered vertically
- « Colorful Diwali Wishes Share Via Whatsapp and Facebook
- « Get City and State by ZipCode Using Google Map Geocoding API
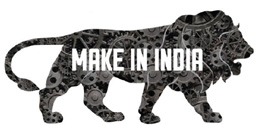