17
04PHP Database Class PDO
PHP database class is to facilitate PHP/MYSQL development. It propose convenient methods and easy way to access the database by reducing your code and reducing your time to write code in a lengthy manner. It makes the programmer to handle CRUD(Create Read Update Delete) operations very easily.It also provides the debugging facilities so that programmer can display the request and resulting table by just adding a parameter to the method of this class. Queries are aitomatically debugged when there is an errror. This php database class is bulid using PDO and BindParams so its SQL injection safe. Try to use this in all your application so that you can finish your work in an easy and simple manner.
Why we have to use PHP database Class?
PHP database class prevent you from writting more code, you can write the code in a single line instead of writing more than 10 line to execute the simple fetch query in PDO.
The first important thing is to configure the database. Follow the below step to cofigure the PHP Database class.
- 1. Go to Db.php
- 2. Edit the $_host, $_user, $_pass, $_db and provide your hostname, username, password and database
Fetch data with PDO
//connect to database try{ $con = new PDO("mysql:host=localhost;dbname=test","root","password"); }catch (PDOException $e){ die($e); } //prepare the query $stmt = $con->prepare("SELECT * FROM table WHERE password=?"); //bind value to prevent from SQL injection $stmt->bindValue(1, "password"); //execute the query $stmt->execute(); //fetch the result $result = $stmt->fetchAll(PDO::FETCH_ASSOC); //display the result print ""; print_r($result); print "";
Fetch data in PHP Database Class
//fetch result $con = Db::fetch("test",array("password" => "password"),array("=")); //display result print_r($con);
Starting a connection
require_once "./class/Db.php"; //include PHP database CLass Db::getConnection();
Checking error
This method check query was successfull or not. It return false when query was successful with not error. Return true when query failedrequire_once "./class/Db.php"; //include PHP database CLass if(Db::getError() == false){ //query was successful }else{ //query was not succesfull }
Inserting a record
Suppose consider the table name user, in there we have to store the username and password.require_once "./class/Db.php"; //include PHP database CLass //insert in user table Db::insert("user",array( "username" => $username, "password" => $password, "country" => $country ));
Updating a Record
In the table name user we have to update the username and password for the id =1.require_once "./class/Db.php"; //include PHP database CLass //insert in user table Db::insert("user",array( "username" => $username, "password" => $password, "country" => $country ));
Deleting a record
Suppose we have to delete the id = 1 from the table user.
require_once "./class/Db.php"; //include PHP database CLass
//delete a record
Db::delete("test",array("id","=",1));
Fetching the record with single condition
Fretch all data from the table where country = India
require_once "./class/Db.php"; //include PHP database CLass
//fetch
$r = Db::fetch("test",array("country" => "India"),array("="));
//display result
print_r($r);
Fetching the record with multiple conditon
Suppose we have to fetch the data with the multiple condition look the below code.
require_once "./class/Db.php";
$result = Db::fetch("user",array(
"email" => "email@gmail.com",
"password" => "password"
),array("=","!="));
print_r($result);
Row Count
To get the number of rows from the table user follow the below code
require_once "./class/Db.php";
$row = Db::rowCount('test');
echo $row;
Close the connection
require_once "./class/Db.php"; //include PHP database CLass
//unset connecton
Db::unsetConnection();
require_once "./class/Db.php"; //include PHP database CLass //delete a record Db::delete("test",array("id","=",1));
Fetching the record with single condition
Fretch all data from the table where country = Indiarequire_once "./class/Db.php"; //include PHP database CLass //fetch $r = Db::fetch("test",array("country" => "India"),array("=")); //display result print_r($r);
Fetching the record with multiple conditon
Suppose we have to fetch the data with the multiple condition look the below code.require_once "./class/Db.php"; $result = Db::fetch("user",array( "email" => "email@gmail.com", "password" => "password" ),array("=","!=")); print_r($result);
Row Count
To get the number of rows from the table user follow the below coderequire_once "./class/Db.php"; $row = Db::rowCount('test'); echo $row;
Close the connection
require_once "./class/Db.php"; //include PHP database CLass //unset connecton Db::unsetConnection();
Please click the download link to get the DB.php file
By Thirumani Raj posted on - 17th Apr 2016
Social Oauth Login
Personalized Map Navigation
Online Image Compression Tool
Image CompressionAdvertisement
Recent Posts
- « Personalized Map Navigation
- « Remjs solves the problem of mobile terminal adaptation
- « Picture centered vertically
- « Colorful Diwali Wishes Share Via Whatsapp and Facebook
- « Get City and State by ZipCode Using Google Map Geocoding API
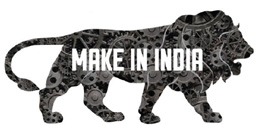